Encapsulation in Object Oriented Programming
Encapsulation is one of the main pillars of object oriented programming (OOPs) in which we bundles the attributes (data) and the functions (methods) that operate on that data within the class. It help in data hiding and abstraction , making our code more organized, secure & reusable.
The following image may be helpful in understanding this concept.
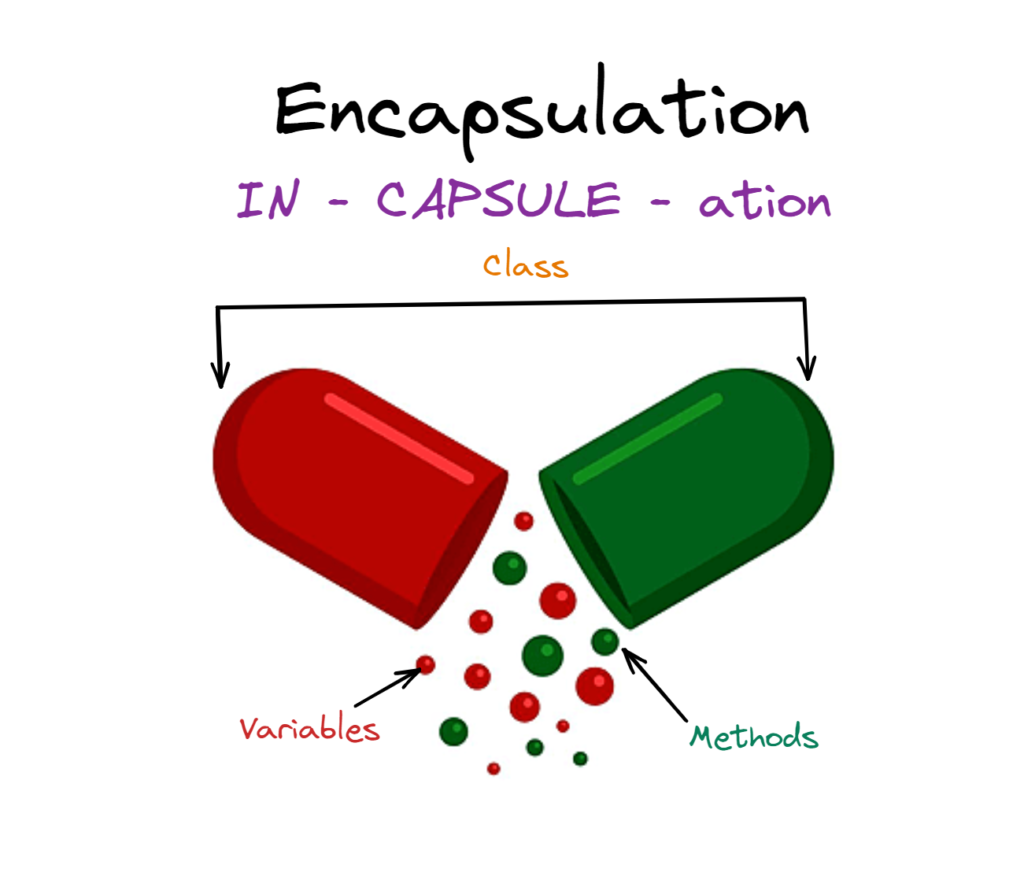
Example
Following is the code example using javaScript.
function Person(name, age) {
let privateName = name;
let privateAge = age;
this.getName = function() {
return privateName;
};
this.getAge = function() {
return privateAge;
};
this.setAge = function(newAge) {
if (newAge >= 0) {
privateAge = newAge;
} else {
console.log("Age cannot be negative.");
}
};
}
let person1 = new Person("Alice", 30);
console.log(person1.getName()); // Output: Alice
console.log(person1.getAge()); // Output: 30
person1.setAge(-5); // Output: Age cannot be negative.
Benefits of Encapsulation
Data Protection/Data Hiding(Security)
Encapsulation is a way of controlling access to the state of an object for objects as it hides them from the outside world by only letting them access through the interface. Using getter and setter methods, we can also control the how the external code interacts with the internal state of an object.
Maintainability
Encapsulation makes code more manageable and refactoring/modifying more stylish, change can be made in the class without affecting the other part of code/program.
Modularity
Encapsulated classes are self contained and can easily be used in different parts of the program/code.
Abstraction
Encapsulation on the other hand gives a more refined way of accessing an object so that a user can only in deed interface with the object and not how it is being implemented.
Implementing Encapsulation
In most OOP supporting programming languages , it is achieved by using access modifiers. These access modifiers control the visibility of class members i.e., attributes and methods.
Example
class Person {
// Private property (using the # symbol)
#name;
constructor(name, age) {
this.#name = name; // private field
this.age = age; // public field
}
// Public method
getDetails() {
return `${this.#name} is ${this.age} years old.`;
}
// Private method (cannot be accessed outside the class)
#getPrivateMessage() {
return `This is a private method.`;
}
// Public method that uses the private method
showPrivateMessage() {
return this.#getPrivateMessage();
}
}
const person = new Person('John Doe', 30);
console.log(person.getDetails()); // "John Doe is 30 years old."
console.log(person.age); // 30 (public property, accessible)
// The following lines will throw an error because #name and #getPrivateMessage are private
// console.log(person.#name);
// console.log(person.#getPrivateMessage());
console.log(person.showPrivateMessage()); // "This is a private method."
Conclusion
Encapsulation supports and promotes the data hiding, security, modularity and abstraction which help us to make our code organized, maintainable and secure , which lead to better software design.